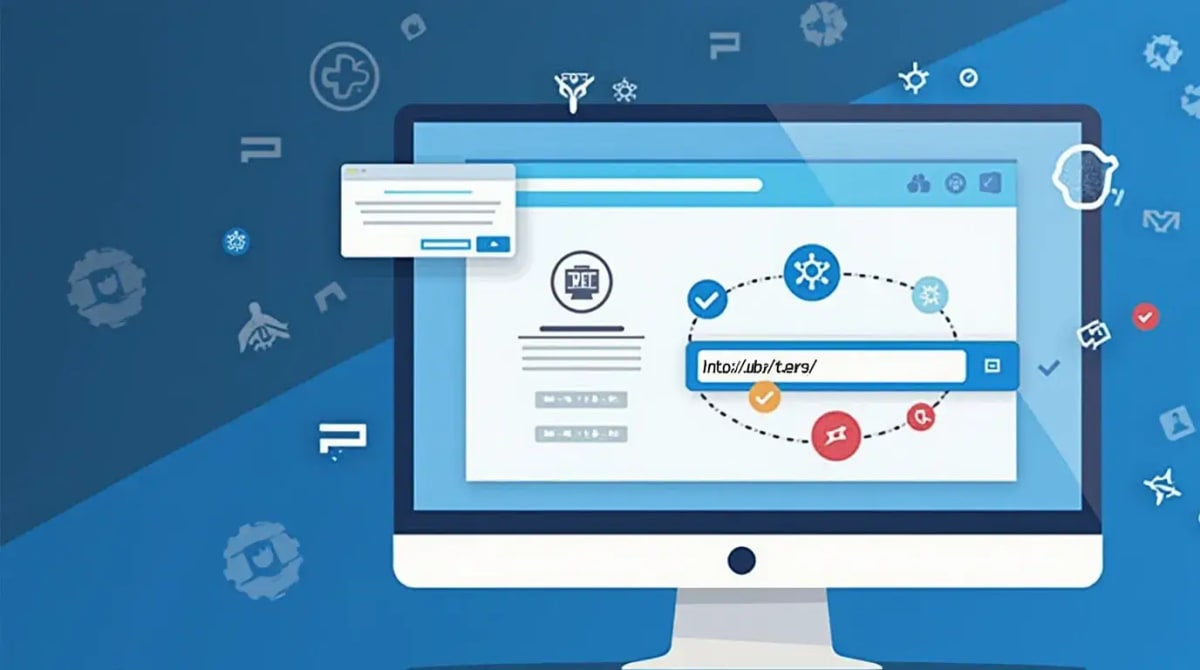
How To Make a Url Shortener
Published on: December 18, 2024
Introduction
In today’s digital world, URLs have become the key to accessing content online. However, long and cumbersome URLs can sometimes be unwieldy, particularly for sharing across social media or in marketing materials. This is where a URL shortener comes into play, condensing long web addresses into shorter, more manageable versions. In this article, we will explore how to create your own URL shortener from scratch, outlining the technical steps and considerations to build a fully functional tool.
What is a URL Shortener?
A URL shortener is a service that converts a long URL into a significantly shorter version. This short URL typically redirects users to the original web address, but its brevity makes it more convenient for sharing, especially in places where character limits are imposed, like Twitter. URL shorteners often come with added features such as click tracking and customizable links.
Popular URL Shortener Services
Some of the most well-known URL shorteners include Bitly
, TinyURL
, and Google URL
Shortener (now defunct). These platforms provide users with easy-to-use interfaces to shorten their links
quickly. However, building your own URL shortener gives you more control over branding and additional features.
How URL Shorteners Work
Behind the Magic: The Process of Shortening a URL
When a user submits a long URL to a shortener, the service generates a unique identifier (usually a random
alphanumeric string). This identifier is then appended to the base URL of the shortener service. For example, a
long URL like https://www.example.com/a-very-long-page-title
might become something like
https://short.ly/abc123
.
Creating Unique, Short Links
The key to a successful URL shortener is ensuring that each shortened link is unique. The system assigns an identifier that is not already in use, typically using a combination of random strings and hashes.
Redirect Mechanism
When a user clicks on the shortened URL, they are redirected to the original, longer URL through an HTTP redirect, which tells the browser to navigate to the new location.
Key Benefits of Using URL Shorteners
Aesthetic and Usability Benefits
Shortened URLs are visually appealing and make links easier to share, particularly in platforms with limited space. For businesses and marketers, they also present a cleaner look that aligns with branding.
Tracking and Analytics
URL shorteners allow for detailed tracking of how often a link is clicked, where the clicks come from, and when the clicks happen. This data is invaluable for understanding audience behavior.
Social Media Optimization
Short URLs are perfect for social media posts, where brevity is essential. They fit neatly into tweets, Facebook posts, and Instagram captions, making them ideal for sharing links.
Basic Components of a URL Shortener
Frontend: User Interface Design
The frontend consists of the interface where users input their long URLs to be shortened. It should be simple and intuitive, with clear fields for entering a URL and a button to generate the shortened link.
Backend: Server Logic and Database
The backend of a URL shortener handles the core functionality, including the logic for creating shortened URLs, storing original URLs, and managing redirections. The backend typically interacts with a database to store and retrieve URL mappings.
Shortening Algorithm
The core of the URL shortener is its algorithm for generating unique short URLs. This could be as simple as creating a random string or implementing a more sophisticated hash-based system to ensure uniqueness.
Choosing the Right Technology Stack
Frontend: HTML, CSS, and JavaScript
For the frontend, basic web technologies like HTML
, CSS
, and JavaScript
are sufficient to create a functional user interface. You may also choose to use frontend frameworks like React
for a more dynamic experience.
Backend: Node.js and Express
For the backend, Node.js
and Express
are popular choices due to their simplicity and
scalability. Express simplifies the process of handling HTTP requests, which is essential for creating a URL
shortener service.
Database Options: MongoDB vs. SQL
For storing URL mappings, a NoSQL database like MongoDB
is a good option for its flexibility and
ease of use. Alternatively, an SQL database like MySQL
can be used if you prefer a relational
structure.
Setting Up the Project Environment
Installing Necessary Software and Dependencies
To get started, you will need to install Node.js
and NPM
(Node Package Manager) to
manage your project’s dependencies. For the database, you’ll also need to set up MongoDB
or a
similar service.
Directory Structure Setup
Create a project folder that includes subfolders for your frontend (frontend/
) and backend
(backend/
). Organize your files to ensure that the server-side code, database models, and frontend
assets are easy to manage.
Initializing the Project
Once the environment is set up, initialize your project using npm init
and install necessary
dependencies such as Express
, MongoDB
, and Mongoose
for database
interaction.
Creating the URL Shortener Backend
Setting Up Express Server
Start by setting up a basic Express
server with routes to handle requests for shortening URLs and
redirecting users. This server will listen for incoming HTTP requests, process them, and send appropriate
responses.
Database Integration and URL Storage
Use MongoDB
(or another database) to store the original URLs and their corresponding short URLs.
You’ll need to define a schema and model to structure this data for easy retrieval and updates.
Creating the Shortening Logic
For the URL shortening functionality, create a function that generates a unique identifier for each long URL. This can be achieved using random string generation or hash functions.
Implementing the URL Redirection Logic
Generating Short URLs
When a user submits a URL, generate a unique short link and store it in the database. This short link should be easy to share and point to the original URL.
Redirecting Users from Short URLs to Original Links
Set up a redirect route on your server to handle requests for shortened URLs. When a user accesses a short URL, the server looks up the corresponding original URL in the database and redirects the user to it.
Handling Expired or Invalid Links
Ensure that your system handles expired or invalid links properly by checking the database for the existence of the original URL before redirecting. If no match is found, return a 404 error.
Building the Frontend for URL Input
Simple User Interface Design
The frontend should provide users with a clean interface to input their URLs. Consider using input fields, a submit button, and a display area to show the shortened URL.
Styling the Frontend
Use CSS or CSS frameworks like Bootstrap or Material-UI to make the interface visually appealing. Ensure that the design is responsive and works across different devices.
Testing and Deployment
Unit Testing the Backend Logic
Before deploying your URL shortener, perform unit testing to ensure that the backend logic works as expected. Use
testing libraries like Jest
or Mocha
to write test cases for your functions.
Deploying the Application
Once everything is working locally, deploy your application to a hosting platform like Heroku
or
Netlify
for easy access and public use.