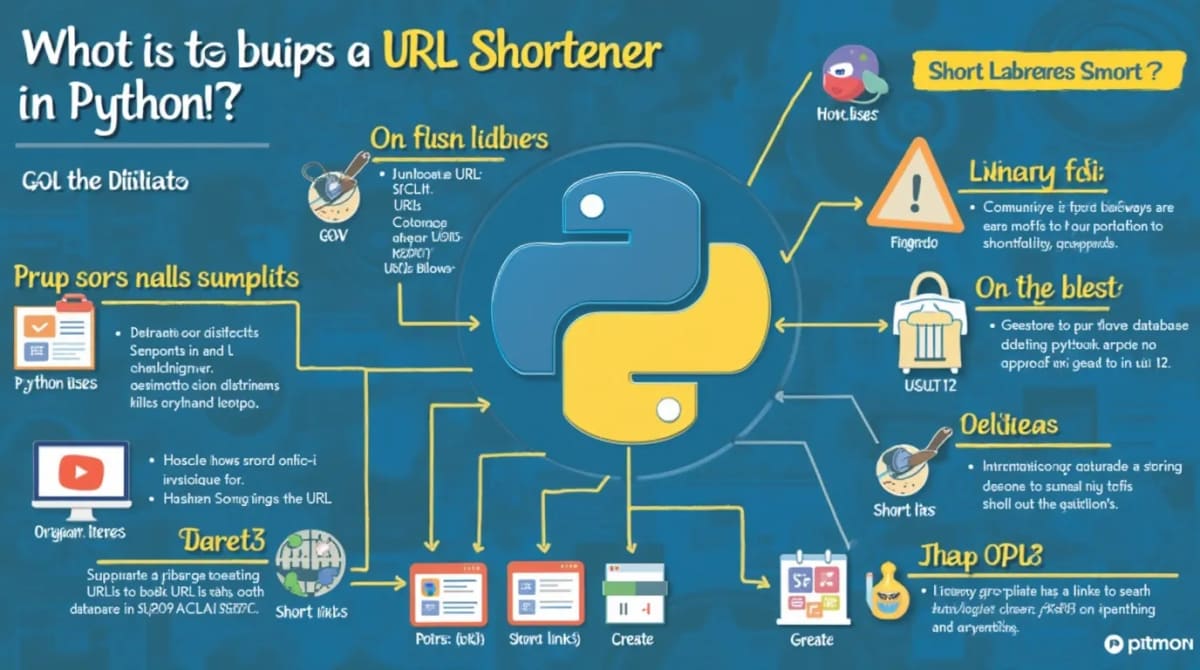
How to Make a URL Shortener in Python
Last updated on: June 28, 2025
Introduction
URL shorteners are a powerful tool that allow you to reduce lengthy and cumbersome web addresses into concise, shareable links. Whether for social media or email, shortening URLs makes sharing more convenient. In this guide, we'll walk you through how to create your own URL shortener in Python, providing you with both the skills and knowledge to develop this practical tool.
The Importance of URL Shorteners
URL shorteners have become essential in the digital age. They make links more manageable, especially when dealing with long, complex URLs. These shortened links are often used in marketing campaigns, social media posts, and QR codes for ease of access.
Why Use Python for Building a URL Shortener?
Python is an excellent choice for this project due to its simplicity, vast library ecosystem, and web framework support. Whether you're a beginner or an experienced developer, Python offers an easy-to-understand syntax and robust tools for building efficient web applications.
Understanding the Basics of URL Shortening
What is a URL Shortener?
A URL shortener is a service that takes a long URL and converts it into a much shorter one. The shorter URL is typically a unique code that, when clicked, redirects the user to the original link.
How Do URL Shorteners Work?
When a user enters a long URL into the shortener, the system generates a unique code and stores it in a database. When someone clicks on the shortened link, the server looks up the code in the database and redirects the user to the original URL.
Setting Up the Python Environment
Installing Python
Before you begin, ensure that you have Python installed on your system. Download the latest version of Python from the official website: python.org/downloads.
Installing Required Libraries
To build the URL shortener, you'll need some essential libraries, such as Flask for web development, SQLite for the database, and some helper tools for generating random strings. Install these by running:
pip install Flask SQLite3
Choosing a Database for Storing URLs
Why Choose SQLite or MongoDB?
SQLite is a simple, lightweight database that is perfect for small applications. However, if you need to scale your application later on, MongoDB might be a better choice. For now, we will stick with SQLite for simplicity.
Setting Up SQLite for Your Project
SQLite comes built-in with Python, so there's no need to install it separately. You'll use it to store both the long URLs and their corresponding shortened versions.
Creating the URL Shortener Logic
Generating a Short URL
The core logic of any URL shortener lies in creating a short, unique identifier. You can use Python's secrets
library to generate a random string of characters as the short URL.
import secrets def generate_short_url(): return secrets.token_urlsafe(6) # Generates a random 6-character string
Redirecting to the Original URL
When someone clicks the shortened link, the system must find the corresponding long URL in the database and redirect the user there. This can be achieved using Flaskās redirect()
method.
Building the Frontend of the URL Shortener
Creating a Simple Web Interface with HTML
For a simple URL shortener, a basic HTML form will suffice. Create a form where users can input their long URL and submit it for shortening.
URL Shortener
Adding CSS for Styling the Interface
Use CSS to enhance the look of your web interface. Keep the design simple, ensuring that it is both functional and aesthetically pleasing.
Implementing URL Validation
Validating User Input
It's important to ensure that the user inputs a valid URL. Use regular expressions to validate the URL format before processing it.
Handling Invalid URLs
If the user enters an invalid URL, prompt them with an error message and ask them to enter a valid URL.
Storing URLs in the Database
Storing Long URLs and Short URLs
After generating the short URL, store both the long and short versions in the database. This allows the system to retrieve the correct long URL when a short link is accessed.
Querying the Database to Retrieve Short URLs
When a short URL is clicked, query the database for the corresponding long URL. If it exists, redirect the user to the original destination.
Adding Expiry for Short URLs
How to Set an Expiration Date for URLs
For extra functionality, you can set an expiration date for each shortened URL, after which the link will no longer work.
Cleaning Up Expired URLs Automatically
Set up a cron job or a scheduled task to clean expired URLs from the database at regular intervals, ensuring the system stays optimized.
Creating a Redirect Mechanism
Writing the Redirect Code in Python
Flask makes it easy to redirect users. Using the redirect()
function, you can automatically send the user to the long URL stored in the database.
Handling 404 Errors Gracefully
Ensure that your application handles 404 errors when a user accesses a non-existent shortened URL, displaying a user-friendly error page.
Implementing Custom Short URL Features
Allowing Users to Choose Custom Short Links
Allow users to create custom short URLs by specifying their preferred short link in the form. Ensure that the chosen name is unique.
Limiting the Length of Custom Short URLs
To avoid excessively long custom links, impose a character limit for user-generated short URLs.
Testing the URL Shortener
Testing with Different URL Formats
Thoroughly test your shortener by using a variety of URL formats to ensure it handles edge cases.
Ensuring the Redirect Works Properly
Test the redirect functionality to ensure it leads users to the correct long URL, even after the link has been shortened.
Deploying the URL Shortener
Hosting the Application on Heroku or AWS
Once your application is ready, deploy it on a platform like Heroku or AWS to make it publicly accessible. Both services offer free tiers for small projects.
Setting Up a Domain for Your Shortener
For a professional touch, you can set up a custom domain for your URL shortener, making it easier for users to remember and share.
Securing the URL Shortener
Using HTTPS for Secure Communication
Always use HTTPS to encrypt communication between users and your server, ensuring their data is safe during interactions.
Preventing Abuse of the Service
Implement measures such as CAPTCHA or rate-limiting to prevent abuse of your URL shortener and ensure its integrity.
Conclusion
Creating a URL shortener in Python is an excellent way to hone your programming skills while building a practical tool. With the basics covered, you can further enhance your shortener by adding analytics, user authentication, or advanced features. This project provides a solid foundation for both beginners and seasoned developers alike.